更新记录
1.2.1(2019-12-31) 下载此版本
- 支持
npm
&yarn
- 支持多组全局配置
- 支持单个请求局部配置
- 支持配置多组请求响应拦截器
- 支持对requestTask进行操作
- 支持多图上传
- 基于typescript开发可能友好的代码提示
- 内置loading拦截器
为了方便开发,自己使用了一段时间,没什么大问题。 有问题或建议及时联系我,谢谢!
平台兼容性
Request
基于uni.request封装的请求对象
- 支持
npm
&yarn
- 支持多组全局配置
- 支持单个请求局部配置
- 支持配置多组请求响应拦截器
- 支持对requestTask进行操作
- 支持多图上传
- 可能友好的代码提示
例子:
// src/utils/request.js
import { Request } from "@wuhz/uni-app-utils";
// 创建实例
const request = Request.getInstance();
// 设置去全局配置
request.setGlobalConfig([
{
baseUrl: "https://hzyun.top",
header: {
"content-type": "application/x-www-form-urlencoded"
},
data: {
store_id: 1
}
},
{
baseUrl: "https://mt.starli.com.cn/?&r=api",
header: {
"content-type": "application/x-www-form-urlencoded"
}
}
]);
// 使用第二组配置
request.setDefaultConfigIndex(1);
// 添加拦截器
request.addInterceptor(
[
config => {
// 返回 抛出异常
return Promise.reject();
// 不返回或其他任意返回值不处理
// 返回 响应函数
return (res) => {
console.log(res);
// 返回 抛出异常
return Promise.reject();
// 无返回值跳过
// 其他返回值会进入下一个拦截器 直至最终输出 此处可对返回值进行修改
// 注意先进后出原则
return res.data
}
}
]
);
export default request;
// src/pages/index
request.get("/hzyun");
对象API
Request.getInstance()
获取Request实例,使用该方法创建实例,为了确保单例,请不要使用new Request()
创建实例。
-
用法:
import { Request } from "@wuhz/uni-app-utils"; const request = Request.getInstance();
实例API
setGlobalConfig( [ ...globalConfig ] )
设置全局配置
-
参数:
{ object } globalConfig 全局配置数组
{ // 开发者服务器接口地址 baseUrl: string; // 请求参数 data: string | object | ArrayBuffer; // 设置请求的 header,header 中不能设置 Referer。 header?: any; // 如果设为json,会尝试对返回的数据做一次 JSON.parse dataType?: string; // 设置响应的数据类型。合法值:text、arraybuffer responseType?: string; // 超时时间,单位 ms 仅支付宝小程序 timeout?: number; //验证 ssl 证书 仅5+App安卓端支持(HBuilderX 2.3.3+) sslVerify?: boolean; }
-
用法:
request.setGlobalConfig( [ { baseUrl: "http://www.hzyun.top", data: { store_id: 1 }; header: { "content-type": "application/x-www-form-urlencoded" }, dataType: "json", responseType: "text", timeout: 30000, sslVerify: true; }, ...{} ] );
setDefaultConfigIndex( index )
设置全局默认使用的配置,默认使用第一组配置。
-
参数:
{ number } index 配置组索引值
-
用法:
request.setDefaultConfigIndex(1)
getRequestTask( name )
获取uni.request返回的requestTask
-
参数:
{ string } name 发送请求时传入的name值
-
用法:
request.getRequestTask("hzyun")
addInterceptor( [ ...interceptor ] )
添加拦截器
先进后出 类似koa
越先进入的请求,响应越后执行。最终执行函数也如此
-
参数:
{ function } interceptor 拦截器函数
/** * @param { object } config 请求配置 * @param { function } addInterceptorFinal 添加最终执行函数 * @return Promise.reject || false || function */ function interceptor(config, addInterceptorFinal) { // 添加此拦截器最终一定会执行的函数 // 此函数要执行成功才会生效,一定要放在return之前 addInterceptorFinal(() => { console.log("final"); }); // 请求拦截 console.log(config); // 返回 Promise.reject 抛出异常 阻止请求 return Promise.reject("错误信息"); // 返回 false 跳过此拦截器 return false // 返回 function 接收到res参数 响应回来的数据 return (res) { // 响应拦截 console.log(res); // 返回 Promise.reject 抛出异常 阻止响应 return Promise.reject("错误信息"); // 返回 false 跳过此响应拦截器 return false } }
-
用法:
request.addInterceptor([interceptor])
get( uri, data, localOptions )
发送get请求
-
参数:
[必传] { string } uri 资源标志符
[可选] { string | object | ArrayBuffer } data 请求参数
-
[可选] { object } localOptions 局部选项
{ // 局部配置 会覆盖与全局配置重复的字段 config?: { // 开发者服务器接口地址 baseUrl: string; // 设置请求的 header,header 中不能设置 Referer。 header?: any; // 如果设为json,会尝试对返回的数据做一次 JSON.parse dataType?: string; // 设置响应的数据类型。合法值:text、arraybuffer responseType?: string; // 超时时间,单位 ms 仅支付宝小程序 timeout?: number; //验证 ssl 证书 仅5+App安卓端支持(HBuilderX 2.3.3+) sslVerify?: boolean; }, // 使用的全局配置索引 默认为第一组 globalConfigIndex?: number; // 为了获取requestTask添加的name标识 name?: string; }
-
用法:
request.get("/hzyun", { money: 1000000000 }, { config: { { baseUrl: "http://www.hzyun.top", data: { store_id: 1 }; header: { "content-type": "application/x-www-form-urlencoded" }, dataType: "json", responseType: "text", timeout: 30000, sslVerify: true; }, }, globalConfigIndex: 1, name: "hzyun" } )
post( uri, data, localOptions )
同get
put( uri, data, localOptions )
同get
delete( uri, data, localOptions )
同get
trace( uri, data, localOptions )
同get
connect( uri, data, localOptions )
同get
options( uri, data, localOptions )
同get
uploadFiles( uri, options, localOptions )
图片上传,支持多图上传
-
参数:
[必传] { string } uri 资源标志符
[可选] { object } options 图片上传选项
-
[可选] { object } localOptions 局部选项
// localOptions 同get options = { // 必传 文件路径 filePath: [], // 可选 formData: {}, // 默认为file name: "file", // 支付宝必传 fileType: "image" | "video" | "audio" }
-
用法:
request.uploadFiles("/hzyun", { filePath: ["http://hanzhaorz.oss-cn-hangzhou.aliyuncs.com/code.jpeg"], name: "file" } )
问题及建议
- 觉得不错就给个star,谢谢啦!
- 有任何问题或者建议可以issue提交或
直接加我微信
,第一时间解决!
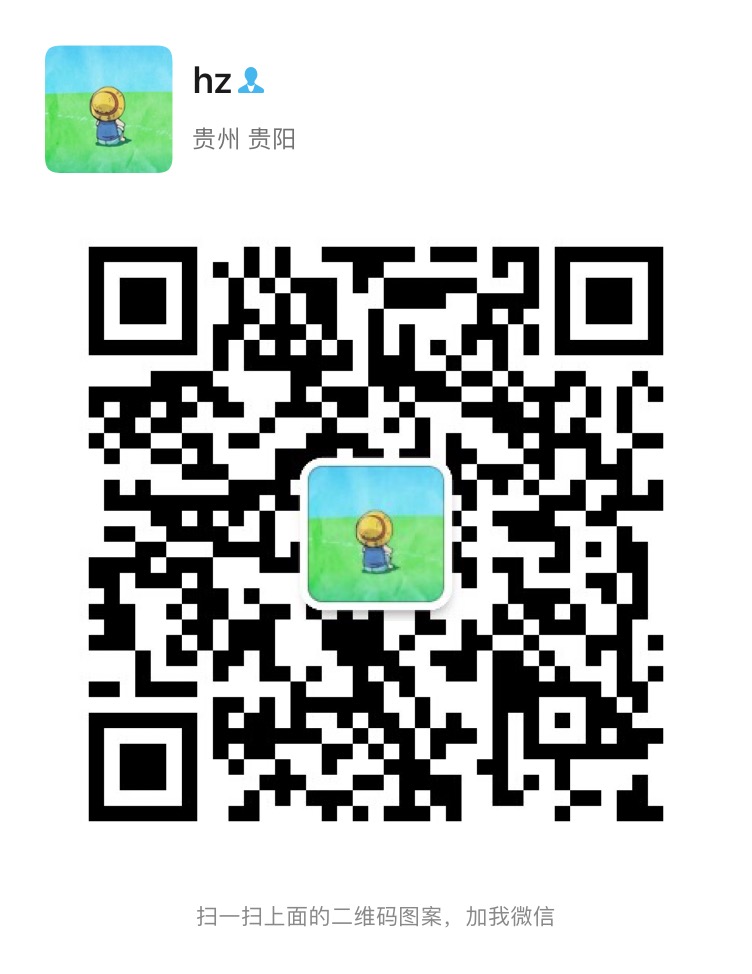